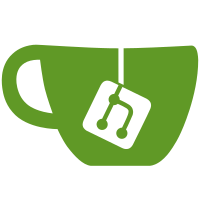
17 changed files with 587 additions and 529 deletions
-
11.gitignore
-
164bin/commands/create-project
-
30bin/commands/install-plugin
-
30bin/commands/install-project-manager
-
12bin/commands/list-projects
-
99bin/commands/remove-project
-
23bin/includes/bash_header
-
214bin/includes/includes
-
165bin/includes/main_functions
-
18bin/includes/project_header
-
301bin/includes/project_manager
-
0bin/postscripts/commands/.keep
-
33etc/.config_template
-
3etc/.customers_template
-
6etc/.project_manager_config_template
-
4etc/.projects_template
-
3etc/.shortnames_template
@ -1,6 +1,11 @@ |
|||||
/.idea |
/.idea |
||||
/.trash |
/.trash |
||||
/bin/postscripts |
|
||||
/data |
|
||||
|
/bin/postscripts/commands/* |
||||
|
/bin/postscripts/plugins/* |
||||
|
/data/* |
||||
/etc/config |
/etc/config |
||||
/etc/projects |
|
||||
|
/etc/projects |
||||
|
/etc/customers |
||||
|
/etc/shortnames |
||||
|
|
||||
|
!/**/.keep |
@ -0,0 +1,23 @@ |
|||||
|
#!/bin/bash |
||||
|
|
||||
|
### DO NOT EDIT THIS FILE |
||||
|
|
||||
|
source "$(cd "$(dirname "${BASH_SOURCE[0]}")" &> /dev/null && pwd)/includes" |
||||
|
|
||||
|
help="$(getParameter "--help" false "$*")" |
||||
|
if [ "$help" == true ] || [ "$1" == "" ] |
||||
|
then |
||||
|
usage |
||||
|
exit |
||||
|
fi |
||||
|
|
||||
|
project_manager_dir="$(cd "$(dirname "${BASH_SOURCE[0]}")/../../" &> /dev/null && pwd)" |
||||
|
|
||||
|
config_file="$(cd "$(dirname "${BASH_SOURCE[0]}")/../.." &> /dev/null && pwd)/etc/config" |
||||
|
if [ -f "$config" ]; then source "$config_file"; fi |
||||
|
projects_file="$(cd "$(dirname "${BASH_SOURCE[0]}")/../.." &> /dev/null && pwd)/etc/projects" |
||||
|
if [ -f "$projects_file" ]; then source "$projects_file"; fi |
||||
|
customers_file="$(cd "$(dirname "${BASH_SOURCE[0]}")/../.." &> /dev/null && pwd)/etc/customers" |
||||
|
if [ -f "$customers_file" ]; then source "$customers_file"; fi |
||||
|
shortnames_file="$(cd "$(dirname "${BASH_SOURCE[0]}")/../.." &> /dev/null && pwd)/etc/shortnames" |
||||
|
if [ -f "$shortnames_file" ]; then source "$shortnames_file"; fi |
@ -0,0 +1,165 @@ |
|||||
|
#!/bin/bash |
||||
|
|
||||
|
### DO NOT EDIT THIS FILE |
||||
|
|
||||
|
if [ true != ${includes_main_functions:-false} ] |
||||
|
then |
||||
|
includes_main_functions=true |
||||
|
|
||||
|
function echoMainTitle { |
||||
|
echo -e "\033[38;5;214m$1\033[0m" |
||||
|
} |
||||
|
|
||||
|
function echoSubTitle { |
||||
|
echo -e "\033[38;5;142m$1\033[0m" |
||||
|
} |
||||
|
|
||||
|
function echoSmall { |
||||
|
echo -e "\033[38;5;240m$1\033[0m" |
||||
|
} |
||||
|
|
||||
|
function echoSelect { |
||||
|
local select="$1" |
||||
|
local message="$2" |
||||
|
echo -e "\033[0;33m$select\033[0m $message" |
||||
|
} |
||||
|
|
||||
|
function echoError { |
||||
|
echo -e "\033[0;91m$1\033[0m" |
||||
|
} |
||||
|
|
||||
|
function echoWarning { |
||||
|
echo -e "\033[0;33m$1\033[0m" |
||||
|
} |
||||
|
|
||||
|
function echoSuccess { |
||||
|
echo -e "\033[0;32m$1\033[0m" |
||||
|
} |
||||
|
|
||||
|
function getParameter { |
||||
|
local name=$1 |
||||
|
local has=$2 |
||||
|
local parameters=($3) |
||||
|
|
||||
|
for i in "${!parameters[@]}" |
||||
|
do |
||||
|
if [ "${parameters[$i]}" == "$name" ] && [ "$has" == true ] |
||||
|
then |
||||
|
((j=i+1)) |
||||
|
echo "${parameters[$j]}" |
||||
|
elif [ "${parameters[$i]}" == "$name" ] |
||||
|
then |
||||
|
echo true |
||||
|
fi |
||||
|
done |
||||
|
} |
||||
|
|
||||
|
function getArgument { |
||||
|
local var=$1 |
||||
|
local errmsg=${2:-"Invalid Argument"} |
||||
|
local allow=${3:-true} |
||||
|
|
||||
|
if [ "$allow" != false ] && [ "$(isAllowed "$var" "$allow")" == false ] |
||||
|
then |
||||
|
echo "$errmsg" >&2 |
||||
|
exit 1 |
||||
|
fi |
||||
|
|
||||
|
echo "$var" |
||||
|
} |
||||
|
|
||||
|
function isAllowed { |
||||
|
local var="$1" |
||||
|
local allow="$2" |
||||
|
local valid=true |
||||
|
|
||||
|
if [ "$allow" == true ] && [ "$var" == "" ] |
||||
|
then |
||||
|
valid=false |
||||
|
elif [ "$allow" != false ] && [ "$allow" != true ] |
||||
|
then |
||||
|
allow=($allow) |
||||
|
local found=false |
||||
|
for i in "${!allow[@]}" |
||||
|
do |
||||
|
if [ "${allow[$i]}" == "$var" ] |
||||
|
then |
||||
|
found=true |
||||
|
fi |
||||
|
done |
||||
|
if [ "$found" != true ] |
||||
|
then |
||||
|
valid=false |
||||
|
fi |
||||
|
fi |
||||
|
|
||||
|
echo $valid |
||||
|
} |
||||
|
|
||||
|
function readConsole { |
||||
|
local message="$1" |
||||
|
local errmsg="$2" |
||||
|
local allow=${3:-false} |
||||
|
local default="$4" |
||||
|
|
||||
|
if [ "$default" != "" ] |
||||
|
then |
||||
|
read -p "$message [default:$default]: " var |
||||
|
else |
||||
|
read -p "$message: " var |
||||
|
fi |
||||
|
|
||||
|
if [ "$var" == "" ] && [ "$default" != "" ] |
||||
|
then |
||||
|
var="$default" |
||||
|
fi |
||||
|
|
||||
|
if [ "$(isAllowed "$var" "$allow")" == false ] |
||||
|
then |
||||
|
echo "$errmsg" >&2 |
||||
|
var=$(readConsole "$message" "$errmsg" "$allow" "$default") |
||||
|
fi |
||||
|
|
||||
|
echo "$var" |
||||
|
} |
||||
|
|
||||
|
function postScript { |
||||
|
local script=$(getArgument "$1" "Usage: postScript \"post_script.sh\"" true) |
||||
|
if [ -f "$script" ] |
||||
|
then |
||||
|
source "$script" |
||||
|
fi |
||||
|
} |
||||
|
|
||||
|
function getConfig { |
||||
|
local env=$(getArgument "$1" "Usage getEnvVar [live|stage|local_live|local_stage] var" "live stage local_live local_stage") |
||||
|
local suffix=$(getArgument "$2" "Usage getEnvVar [live|stage|local_live|local_stage] var" true) |
||||
|
echo "$(eval "echo \"\$${env}_$suffix\"")" |
||||
|
} |
||||
|
|
||||
|
function sedEscape { |
||||
|
echo "$1" | sed -r 's/([\$\.\*\/\[\\^])/\\\1/g' | sed 's/[]]/\[]]/g' |
||||
|
} |
||||
|
|
||||
|
function confirm { |
||||
|
confirm="$(readConsole "Should i execute? [y,n]" "Invalid selection" "y n")" |
||||
|
|
||||
|
if [ "$confirm" == "n" ] |
||||
|
then |
||||
|
echo |
||||
|
echoError "Aborting" |
||||
|
echo |
||||
|
exit |
||||
|
fi |
||||
|
} |
||||
|
|
||||
|
function getSudoPassword { |
||||
|
if [ "${project_manager_sudo_password}" == "" ] |
||||
|
then |
||||
|
echo -n "Sudo Password:" |
||||
|
read -s project_manager_sudo_password |
||||
|
fi |
||||
|
echo project_manager_sudo_password |
||||
|
} |
||||
|
|
||||
|
fi |
@ -1,18 +0,0 @@ |
|||||
#!/bin/bash |
|
||||
|
|
||||
### DO NOT EDIT THIS FILE |
|
||||
|
|
||||
source "$project_manager_dir/bin/includes/includes" |
|
||||
|
|
||||
help="$(getParameter "--help" false "$*")" |
|
||||
if [ "$help" == true ] || [ "$1" == "" ] |
|
||||
then |
|
||||
usage |
|
||||
exit |
|
||||
fi |
|
||||
|
|
||||
shortname="$(getArgument "$1" "$(usage)" true)" |
|
||||
project="$(getProjectFromShortname "$shortname")" |
|
||||
customer="$(getCustomerFromShortname "$shortname")" |
|
||||
loadProjectConfig "$shortname" |
|
||||
app_dir="$project_manager_dir/data/$customer/$project" |
|
@ -0,0 +1,301 @@ |
|||||
|
#!/bin/bash |
||||
|
|
||||
|
### DO NOT EDIT THIS FILE |
||||
|
|
||||
|
if [ true != ${includes_project_manager:-false} ] |
||||
|
then |
||||
|
includes_project_manager=true |
||||
|
|
||||
|
function getProjectFromShortname { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
for i in "${!project_manager_shortnames[@]}" |
||||
|
do |
||||
|
if [ "${project_manager_shortnames[$i]}" == "$shortname" ] |
||||
|
then |
||||
|
echo "${project_manager_projects[$i]}" |
||||
|
fi |
||||
|
done |
||||
|
} |
||||
|
|
||||
|
function getCustomerFromShortname { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
for i in "${!project_manager_shortnames[@]}" |
||||
|
do |
||||
|
if [ "${project_manager_shortnames[$i]}" == "$shortname" ] |
||||
|
then |
||||
|
echo "${project_manager_customers[$i]}" |
||||
|
fi |
||||
|
done |
||||
|
} |
||||
|
|
||||
|
function loadProjectConfig |
||||
|
{ |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local customer="$(getCustomerFromShortname "$shortname")" |
||||
|
local project="$(getProjectFromShortname "$shortname")" |
||||
|
|
||||
|
if [ "$customer" == "" ] || [ "$project" == "" ] |
||||
|
then |
||||
|
echo |
||||
|
echoError "Could not load project with name: $shortname" |
||||
|
echo |
||||
|
exit |
||||
|
fi |
||||
|
|
||||
|
local path="$project_manager_dir/data/$customer/$project" |
||||
|
source "$path/etc/config" |
||||
|
} |
||||
|
|
||||
|
function loadPluginConfig |
||||
|
{ |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local customer="$(getCustomerFromShortname "$shortname")" |
||||
|
local project="$(getProjectFromShortname "$shortname")" |
||||
|
|
||||
|
if [ "$customer" == "" ] || [ "$project" == "" ] |
||||
|
then |
||||
|
echo |
||||
|
echoError "Could not load project with name: $shortname" |
||||
|
echo |
||||
|
exit |
||||
|
fi |
||||
|
|
||||
|
local path="$project_manager_dir/data/$customer/$project" |
||||
|
local plugin_path="$project_manager_dir/plugins" |
||||
|
local plugins="$(ls -t "$plugin_path")" |
||||
|
|
||||
|
for plugin in ${plugins[*]} |
||||
|
do |
||||
|
if [ -f "$path/etc/plugins/$plugin/config" ] |
||||
|
then |
||||
|
source "$path/etc/plugins/$plugin/config" |
||||
|
fi |
||||
|
done |
||||
|
} |
||||
|
|
||||
|
function makeProjectManagerDirectories { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local customer="$(getArgument "$2")" |
||||
|
local project="$(getArgument "$3")" |
||||
|
|
||||
|
path="$project_manager_dir/data/$customer/$project" |
||||
|
|
||||
|
if [ ! -d "$path" ]; then mkdir -p "$path"; fi |
||||
|
if [ ! -d "$path/.ssh" ]; then mkdir "$path/.ssh"; fi |
||||
|
if [ ! -d "$path/backup" ]; then mkdir "$path/backup"; fi |
||||
|
if [ ! -d "$path/backup/database" ]; then mkdir "$path/backup/database"; fi |
||||
|
if [ ! -d "$path/bin" ]; then mkdir "$path/bin"; fi |
||||
|
if [ ! -d "$path/bin/postscripts" ]; then mkdir "$path/bin/postscripts"; fi |
||||
|
if [ ! -d "$path/bin/postscripts/plugins" ]; then mkdir "$path/bin/postscripts/plugins"; fi |
||||
|
if [ ! -d "$path/bin/includes" ]; then mkdir "$path/bin/includes"; fi |
||||
|
if [ ! -d "$path/bin/includes/plugins" ]; then mkdir "$path/bin/includes/plugins"; fi |
||||
|
if [ ! -d "$path/etc" ]; then mkdir "$path/etc"; fi |
||||
|
if [ ! -d "$path/etc/plugins" ]; then mkdir "$path/etc/plugins"; fi |
||||
|
if [ ! -d "$path/shared" ]; then mkdir "$path/shared"; fi |
||||
|
if [ ! -d "$path/shared/live" ]; then mkdir "$path/shared/live"; fi |
||||
|
if [ ! -d "$path/shared/stage" ]; then mkdir "$path/shared/stage"; fi |
||||
|
if [ ! -d "$path/var" ]; then mkdir "$path/var"; fi |
||||
|
if [ ! -d "$path/var/tmp" ]; then mkdir "$path/var/tmp"; fi |
||||
|
if [ ! -d "$path/var/latest" ]; then mkdir "$path/var/latest"; fi |
||||
|
} |
||||
|
|
||||
|
function makeWorkspaceDirectories { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local customer="$(getArgument "$2")" |
||||
|
local project="$(getArgument "$3")" |
||||
|
|
||||
|
if [ ! -d "$project_manager_workspaces_dir/$customer/$project" ]; then mkdir -p "$project_manager_workspaces_dir/$customer/$project"; fi |
||||
|
} |
||||
|
|
||||
|
function addProjectConfig { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local customer="$(getArgument "$2")" |
||||
|
local project="$(getArgument "$3")" |
||||
|
|
||||
|
_addProject "$project" |
||||
|
_addCustomer "$customer" |
||||
|
_addShortname "$shortname" |
||||
|
} |
||||
|
|
||||
|
function hasShortname { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
|
||||
|
for i in "${!project_manager_shortnames[@]}" |
||||
|
do |
||||
|
if [ "${project_manager_shortname[$i]}" == "$shortname" ] |
||||
|
then |
||||
|
echo true |
||||
|
fi |
||||
|
done |
||||
|
echo false |
||||
|
} |
||||
|
|
||||
|
function hasProject { |
||||
|
local project="$(getArgument "$1")" |
||||
|
|
||||
|
for i in "${!project_manager_projects[@]}" |
||||
|
do |
||||
|
if [ "${project_manager_projects[$i]}" == "$project" ] |
||||
|
then |
||||
|
echo true |
||||
|
fi |
||||
|
done |
||||
|
echo false |
||||
|
} |
||||
|
|
||||
|
function _addShortname { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local hasShortname="$(hasShortname "$shortname")" |
||||
|
|
||||
|
if [ "$hasShortname" == true ] |
||||
|
then |
||||
|
echo |
||||
|
echoError "Shortname already exists" |
||||
|
echo |
||||
|
exit |
||||
|
fi |
||||
|
|
||||
|
local string=''; |
||||
|
for i in "${!project_manager_shortnames[@]}" |
||||
|
do |
||||
|
string+="$(echo -e "\n\t'${project_manager_shortnames[$i]}'")" |
||||
|
done |
||||
|
string+="$(echo -e "\n\t'$shortname'")" |
||||
|
_writeShortnames "$string" |
||||
|
} |
||||
|
|
||||
|
function _addProject { |
||||
|
local project="$(getArgument "$1")" |
||||
|
local hasProject="$(hasProject "$project")" |
||||
|
|
||||
|
if [ "$hasProject" == true ] |
||||
|
then |
||||
|
echo |
||||
|
echoError "Project already exists" |
||||
|
echo |
||||
|
exit |
||||
|
fi |
||||
|
|
||||
|
local string=''; |
||||
|
for i in "${!project_manager_projects[@]}" |
||||
|
do |
||||
|
string+="$(echo -e "\n\t'${project_manager_projects[$i]}'")" |
||||
|
done |
||||
|
string+="$(echo -e "\n\t'$project'")" |
||||
|
_writeProjects "$string" |
||||
|
} |
||||
|
|
||||
|
function _addCustomer { |
||||
|
local customer="$(getArgument "$1")" |
||||
|
|
||||
|
local string=''; |
||||
|
for i in "${!project_manager_customers[@]}" |
||||
|
do |
||||
|
string+="$(echo -e "\n\t'${project_manager_customers[$i]}'")" |
||||
|
done |
||||
|
string+="$(echo -e "\n\t'$customer'")" |
||||
|
_writeCustomers "$string" |
||||
|
} |
||||
|
|
||||
|
function _writeShortnames { |
||||
|
local string="$(getArgument "$1")" |
||||
|
cat <<- EOF > "$project_manager_dir/etc/shortnames" |
||||
|
#!/bin/bash |
||||
|
|
||||
|
project_manager_shortnames=($string |
||||
|
) |
||||
|
EOF |
||||
|
} |
||||
|
|
||||
|
function _writeCustomers { |
||||
|
local string="$(getArgument "$1")" |
||||
|
cat <<- EOF > "$project_manager_dir/etc/customers" |
||||
|
#!/bin/bash |
||||
|
|
||||
|
project_manager_customers=($string |
||||
|
) |
||||
|
EOF |
||||
|
} |
||||
|
|
||||
|
function _writeProjects { |
||||
|
local string="$(getArgument "$1")" |
||||
|
cat <<- EOF > "$project_manager_dir/etc/projects" |
||||
|
#!/bin/bash |
||||
|
|
||||
|
project_manager_projects=($string |
||||
|
) |
||||
|
EOF |
||||
|
} |
||||
|
|
||||
|
function removeProjectConfig { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
local pos="$(getProjectConfigPosition "$shortname")" |
||||
|
|
||||
|
_removeShortname "$pos" |
||||
|
_removeCustomer "$pos" |
||||
|
_removeProject "$pos" |
||||
|
} |
||||
|
|
||||
|
function getProjectConfigPosition { |
||||
|
local shortname="$(getArgument "$1")" |
||||
|
|
||||
|
for i in "${!project_manager_shortnames[@]}" |
||||
|
do |
||||
|
if [ "${$project_manager_shortnames[$i]}" == "$shortname" ] |
||||
|
then |
||||
|
((ret=i+1)) |
||||
|
return "$ret" |
||||
|
fi |
||||
|
done |
||||
|
return 0 |
||||
|
} |
||||
|
|
||||
|
function _removeShortname { |
||||
|
local pos="$(getArgument "$1")" |
||||
|
|
||||
|
local string=''; |
||||
|
for i in "${!project_manager_shortnames[@]}" |
||||
|
do |
||||
|
((nr=i+1)) |
||||
|
if [ "$pos" == "$nr" ] |
||||
|
then |
||||
|
continue |
||||
|
fi |
||||
|
string+="$(echo -e "\n\t'${project_manager_shortnames[$i]}'")" |
||||
|
done |
||||
|
_writeShortnames "$string" |
||||
|
} |
||||
|
|
||||
|
function _removeProjects { |
||||
|
local pos="$(getArgument "$1")" |
||||
|
|
||||
|
local string=''; |
||||
|
for i in "${!project_manager_projects[@]}" |
||||
|
do |
||||
|
((nr=i+1)) |
||||
|
if [ "$pos" == "$nr" ] |
||||
|
then |
||||
|
continue |
||||
|
fi |
||||
|
string+="$(echo -e "\n\t'${project_manager_projects[$i]}'")" |
||||
|
done |
||||
|
_writeProjects "$string" |
||||
|
} |
||||
|
|
||||
|
function _removeCustomer { |
||||
|
local pos="$(getArgument "$1")" |
||||
|
|
||||
|
local string=''; |
||||
|
for i in "${!project_manager_customers[@]}" |
||||
|
do |
||||
|
((nr=i+1)) |
||||
|
if [ "$pos" == "$nr" ] |
||||
|
then |
||||
|
continue |
||||
|
fi |
||||
|
string+="$(echo -e "\n\t'${project_manager_customers[$i]}'")" |
||||
|
done |
||||
|
_writeCustomers "$string" |
||||
|
} |
||||
|
|
||||
|
fi |
@ -1,41 +1,24 @@ |
|||||
#!/bin/bash |
#!/bin/bash |
||||
|
|
||||
### GENERAL |
|
||||
|
|
||||
app_customer='' |
|
||||
app_project='' |
|
||||
app_project_manager_dir='' |
|
||||
|
|
||||
### STAGE |
|
||||
|
|
||||
# stage domain |
|
||||
stage_domain='' |
|
||||
|
### LOCAL STAGE ENV |
||||
|
|
||||
# url to stage |
# url to stage |
||||
stage_url='' |
|
||||
|
local_stage_url='' |
||||
|
|
||||
# The httpdocs folder on your server |
# The httpdocs folder on your server |
||||
stage_httpdocs_path='' |
|
||||
|
local_stage_httpdocs_path='' |
||||
|
|
||||
### LIVE |
|
||||
|
|
||||
# live domain |
|
||||
live_domain='' |
|
||||
|
### LOCAL LIVE ENV |
||||
|
|
||||
# url to live |
# url to live |
||||
live_url='' |
|
||||
|
local_live_url='' |
||||
|
|
||||
# The httpdocs folder on your server |
# The httpdocs folder on your server |
||||
live_httpdocs_path='' |
|
||||
|
|
||||
### LOCAL |
|
||||
|
|
||||
# url to your project on the local machine |
|
||||
local_url='' |
|
||||
|
local_live_httpdocs_path='' |
||||
|
|
||||
### POST SCRIPT |
|
||||
|
### POSTSCRIPTS |
||||
|
|
||||
app_dir='$app_project_manager_dir/data/$customer/$project' |
|
||||
|
app_dir= |
||||
if [ -f '$app_dir/etc/post_config.sh' ] |
if [ -f '$app_dir/etc/post_config.sh' ] |
||||
then |
then |
||||
source '$app_dir/etc/post_config.sh' |
source '$app_dir/etc/post_config.sh' |
||||
|
@ -0,0 +1,3 @@ |
|||||
|
#!/bin/bash |
||||
|
|
||||
|
project_manager_customers=() |
@ -1,5 +1,5 @@ |
|||||
#!/bin/bash |
#!/bin/bash |
||||
|
|
||||
workspaces_dir='' |
|
||||
project_manager_dir='' |
|
||||
|
|
||||
|
project_manager_workspaces_dir='' |
||||
|
project_manager_local_apache_httpdocs='' # leave empty if you don't want to use |
||||
|
project_manager_sudo_password='' # leave empty to fetch on call |
@ -1,5 +1,3 @@ |
|||||
#!/bin/bash |
#!/bin/bash |
||||
|
|
||||
projects=() |
|
||||
shortnames=() |
|
||||
customers=() |
|
||||
|
project_manager_projects=() |
@ -0,0 +1,3 @@ |
|||||
|
#!/bin/bash |
||||
|
|
||||
|
project_manager_shortnames=() |
Write
Preview
Loading…
Cancel
Save
Reference in new issue